Objective Redux
Redux made better, objectively.
Make organizing and managing your Redux store easy.
Version 1.0 now available!
Meet your new Redux API
Install
npm install --save redux redux-saga objective-redux
Setup (for React)
import React from 'react';
import ReactDOM from 'react-dom';
import { ObjectiveStoreProvider, ObjectiveStore } from 'objective-redux';
import App from './app';
export const objectiveStore = new ObjectiveStore();
ReactDOM.render(
<ObjectiveStoreProvider objectiveStore={objectiveStore}>
<App />
</ObjectiveStoreProvider>,
document.getElementById('root')
);
Example Slice
import { StateController } from 'objective-redux';
const initialState = { isOn: false };
export class SwitchStateController extends StateController {
constructor() {
super(initialState);
}
static getName() {
return 'switch';
}
setSwitch = this.createReducingAction(
(state, isOn) => ({ isOn })
);
}
SwitchStateController.getInstance(objectiveStore).setSwitch(true);
Start using it now
You can read the full documentation for more detailed information, along with examples.
In addition, you can take a look at the example apps in the project’s GitHub repository.
Why use Objective Redux?
Drop the boilerplate code
Actions are a thing of the past— among other things
Object Redux largely removes the need for action names, actions, switch-statement-reducers, selectors, and dispatching. You just need to write the mutating functions. Objective Redux can take it from there.
// Define your mutation and forget about the rest.
myAction = this.createReducingAction(
(state, payload) => ({
...state,
value: payload.value,
})
);
Easy Debugging
No more global searches for action names
Using Objective Redux, your editor knows exactly where to find everything. That means you get intellisense, jump to definition, and more. Plus, your actions and reducer will never get out-of-sync.
Powerful code splitting and lazy loading
Get the pieces of state you need, when you need them
Stop wiring-up your reducers and sagas manually. And, for that matter, stop using large middleware package to help. Objective Redux will take care of it for you, and it will do it on demand, dynamically, at runtime. Your store no longer needs to know about what’s in it, leaving you free to move parts around as needed.
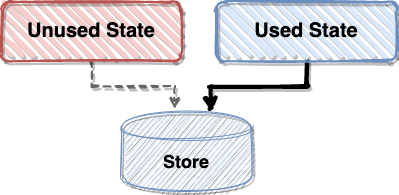
You can even use the pre-dispatch hook to load bundles when an action is fired. This allows you to fire actions that target controllers that haven’t been downloaded, yet.
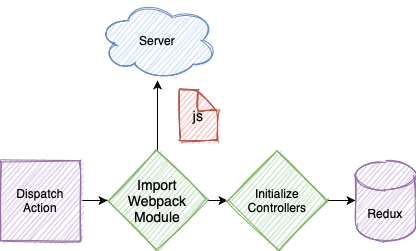
See the Code Splitting and Lazy Loading topics in the documentation for more.
Organize your state
One slice, one object
Each controller class represents a slice, giving an intuitive way for developers to look at and conceptualize the state.
A slice of state never needs to know about what other slices are doing or how they’re organized.
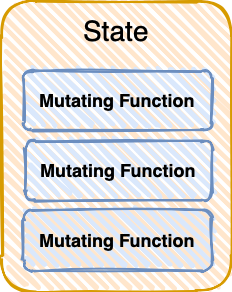
Keep your dependencies simple
No direct dependencies
Objective Redux only requires peer dependencies on Redux and React.
Optionally, you can also add Redux-Saga for StatelessControllers.
Keep your bundle small
Lots of features, one small package
Objective Redux replaces many of the packages you’re already using.
For example, instead of React-Redux + Redux-Injectors + Redux-Toolkit
you can simply use Objective Redux
Bundle sizes vary based on how much of the package is unused and how effectively your bundler can remove the unused portions.
Compatible with React-Redux
Migrate over time
You can use Objective Redux and React-Redux together. The ObjectiveStore is a decorated Redux store object and can be used to dispatch
, subscribe
, getState
, and even replaceReducer
. Simply pass the ObjectiveStore to the React-Redux provider and use it normally.
See the Use with React-Redux topic in the documentation for more.
Multiple ways to connect
Inject properties or use hooks
You can connect your components to Objective Redux to inject props from the store. Or, skip the connection process and use React hooks, instead.